Page
Create an Express.js application
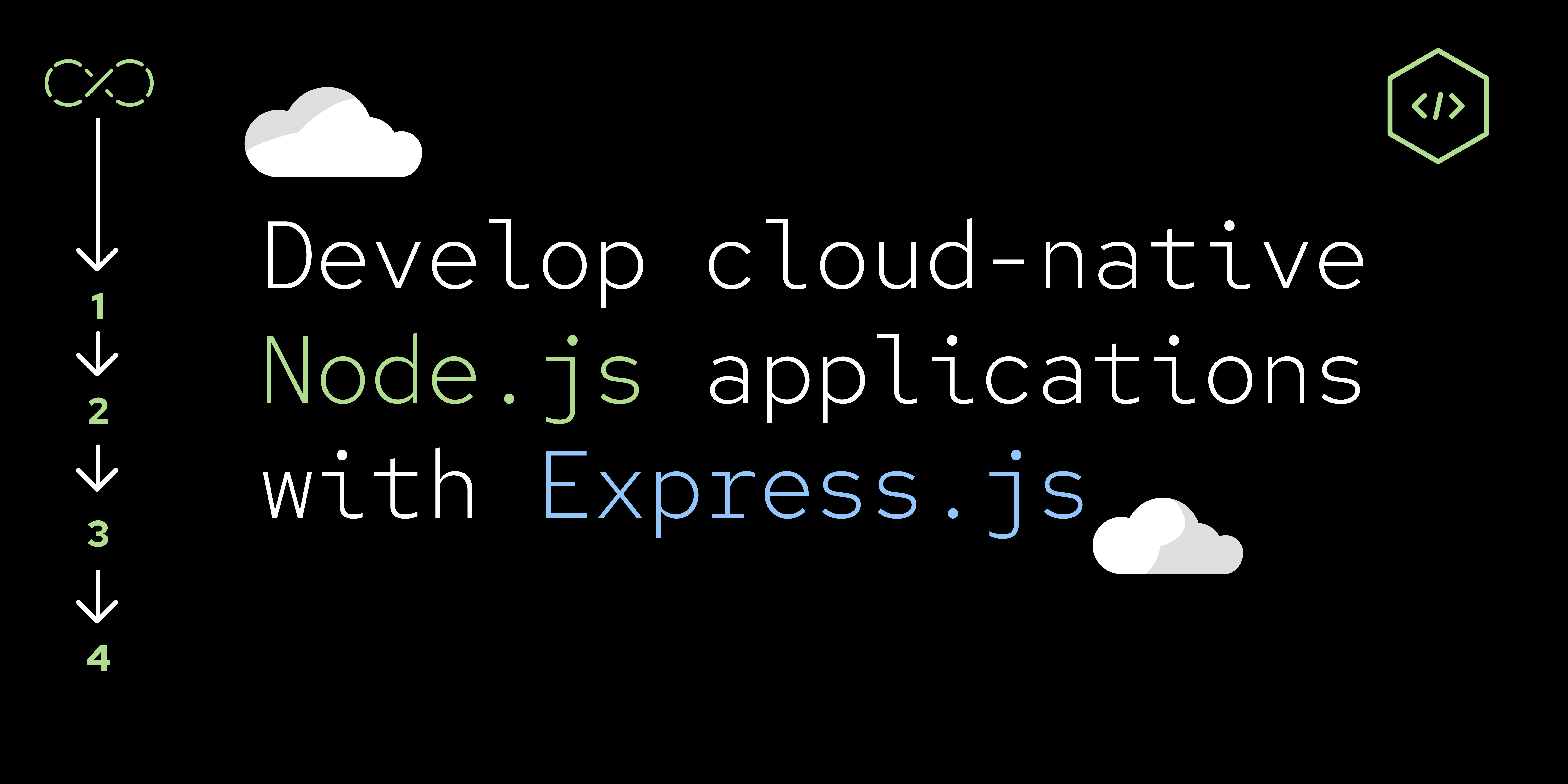
This lesson covers the process of creating a basic Express.js application. Express.js is a popular web server framework for Node.js.
In order to get full benefit from taking this lesson, you need:
- An environment where you can install and run a Node.js application.
In this lesson, you will:
- Create an Express.js application.
- Install a Node.js module for default HTTP headers.
- Install a Node.js module for logging.
Set up the environment
In order to create an Express.js application, the first step is to set up a development environment. This environment will allow you to install all the modules you need to get started with Node.js for cloud-native development.
First, create a directory to host your project:
mkdir nodeserver
cd nodeserver
Next, initialize your project with npm and install the Express.js module:
npm init --yes
npm install express
You'll also install the Helmet module. Helmet is a middleware that you can use to set some sensible default headers on our HTTP requests.
npm install helmet
It is important to add effective logging to your Node.js applications to facilitate observability so that you can understand what is happening in your application. The NodeShift Reference Architecture for Node.js recommends using Pino, a JSON-based logger.
npm install pino
Create your Express.js application
The next step is to start writing the code that will be your application. The first thing to do is create a file named server.js.
Add the following code to server.js to produce an Express.js server that responds on the / route with Hello, World!
.
const express = require('express');
const helmet = require('helmet');
const pino = require('pino')();
const PORT = process.env.PORT || 8080;
const app = express();
app.use(helmet());
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(PORT, () => {
pino.info(`Server listening on port ${PORT}`);
});
Now you can start your application and test that it is working locally.
npm start
> nodeserver@1.0.0 start /private/tmp/nodeserver
> node server.js
{"level":30,"time":1622040801251,"pid":21934,"hostname":"bgriggs-mac","msg":"Server listening on port 8080"}
Navigate to http://localhost:8080 and you should see the server respond with 'Hello, World!'. You can stop your server by entering Ctrl + C in your terminal window.
And that’s it! You have now created an Express.js application, complete with Node.js modules for HTTP headers and logging.
In the next lesson, you will add health checks to your application so your application can be monitored using cloud deployment technology, should the application fail.