Page
Getting to know LLamaIndex.ts with Node.js
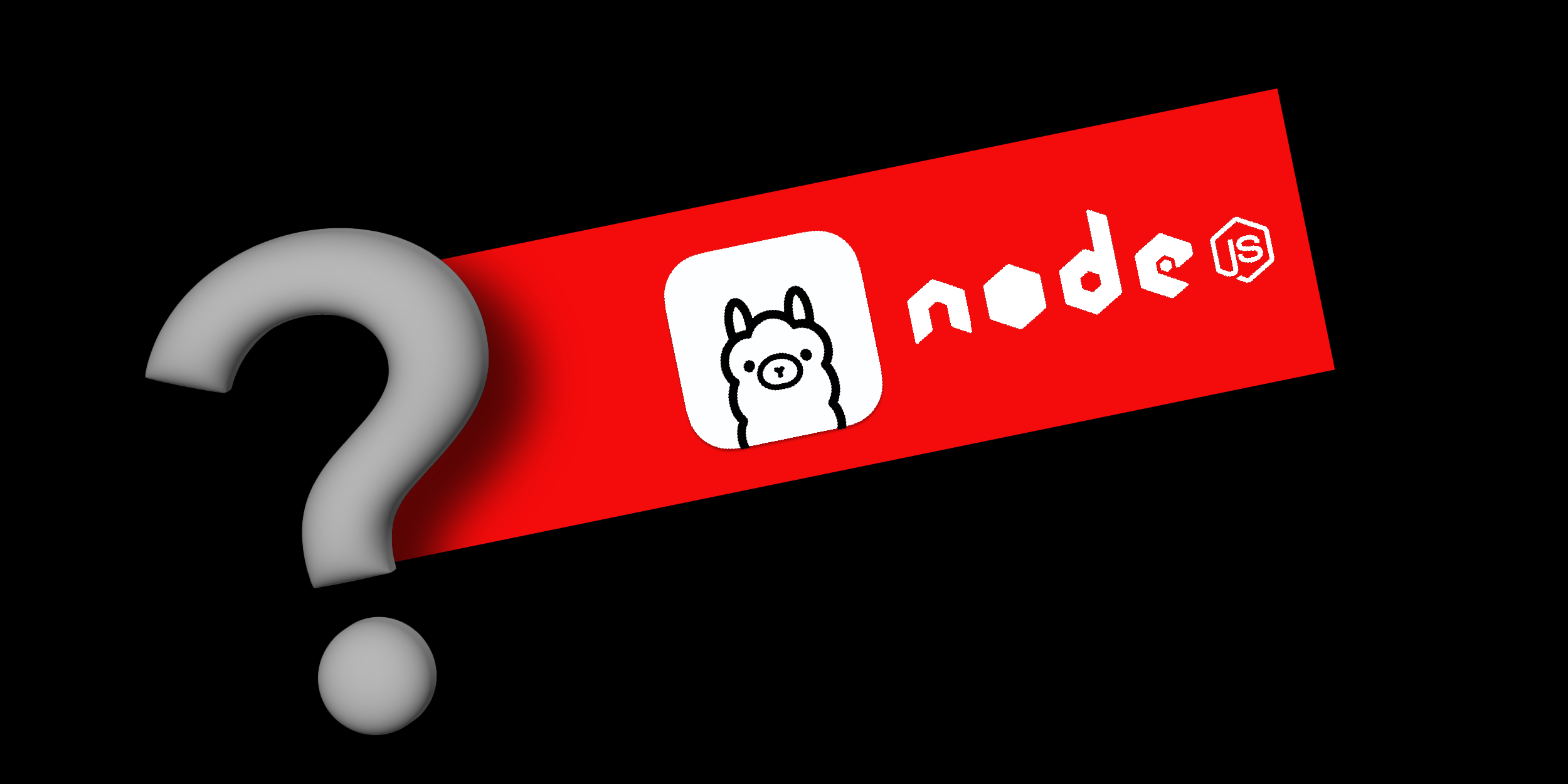
In order to get full benefit from taking this lesson, you need:
- The previous installation of Ollama and Node.js from Lesson 2
In this lesson, you will:
- Review a simple application built with LLamaIndex.ts which asks an LLM a question running on Ollama
- Run the simple application and observe and compare the output to the earlier application using Langchain.js.
Setting up the environment
Change into the lesson-2 directory with
cd ../lesson-2
Install the dependencies for the example with:
npm install
This will install LLamaindex.ts as well as related dependencies.
Running the basic LLamaIndex.ts example
First we’ll start by looking at a basic example using LLamaIndex to ask an LLM a question which Is in llamaindex-ollama.js.
Note: that as for all of the examples you need to modify the Ollama configuration to match how you are running it. Either remove “config: { host: "http://10.1.1.39:11434" },” if you are running locally or configure it to match the IP of the machine you are running it on.
import {
Ollama,
SimpleChatEngine,
} from "llamaindex"
////////////////////////////////
// GET THE MODEL
const llm = new Ollama({
config: { host: "http://10.1.1.39:11434" },
model: "mistral", // Default value
});
////////////////////////////////
// CREATE THE ENGINE
const chatEngine = new SimpleChatEngine({ llm });
////////////////////////////////
// ASK QUESTION
const input = 'should I use npm to start a Node.js application';
console.log(new Date());
const response = await chatEngine.chat({
message: `Answer the following question if you don't know the answer say so: Question: ${input}`
});
console.log(response.response);
console.log(new Date());
In this case we’ve kept it simple and we get the LLM directly instead of using a getModel() function but you can see the code to get the LLM is similar to how it was done with Langchain.js and the same parameters (although with different names) are passed in.
////////////////////////////////
// GET THE MODEL
const llm = new Ollama({
config: { host: "http://10.1.1.39:11434" },
model: "mistral", // Default value
});
We used the simplest LLamaIndex class available to ask questions, SimpleChatEngine, so we needed to substitute the question into the prompt ourselves:
const response = await chatEngine.chat({
message: `Answer the following question if you don't know the answer say so: Question: ${input}`
});
Now run the program with:
node llamaindex-ollama.mjs
You should see an output something like this:
2024-06-10T21:08:46.869Z
Yes, it is common to use npm (Node Package Manager) when starting a Node.js application. npm provides access to a vast number of libraries and tools that can help streamline development, testing, and deployment processes for Node.js projects. You can initialize your project with `npm init`, install dependencies with `npm install`, and start the application using the appropriate command (e.g., `node app.js` or another command based on your project's package.json file).
There are alternatives to npm, such as Yarn and pnpm, but they are also compatible with Node.js projects and offer similar functionalities. Ultimately, the choice between these tools depends on your specific needs and preferences.
2024-06-10T21:08:50.289Z
While answers from the LLM vary, even when exactly the same question is asked, running a number of times you should find that the answers using the LLamaIndex version will look similar to those running with Langchain.js which is expected as they are both just fronting the same LLM running under Ollama.
Just like with Langchain.js we should be able to switch relatively easily between how our LLM is hosted, with LlamaIndex supporting a number of different options:
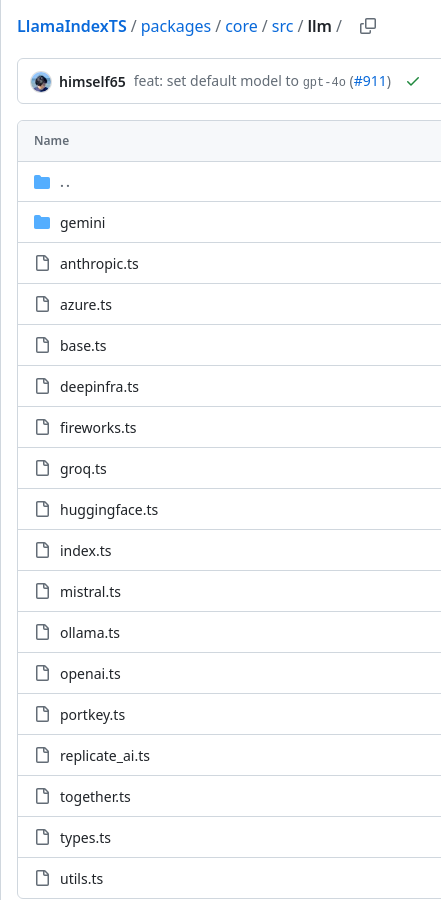
Similar to Langchain.js, LLamaIndex also provides APIs to make it easier to do Retrieval Automated Generation (RAG) and to incorporate data into queries. There is a short example of doing this on the main README.md. Since this is not the focus of this learning path we’ll leave it up to you to explore that further if you are interested.
In this lesson we ran a simple program built using LLamaIndex.TS and Node.js. In the next lesson we will dive into a more complex application that provides functions the LLM can call when needed in order to better answer questions.